Accelerate C++ Chapter01
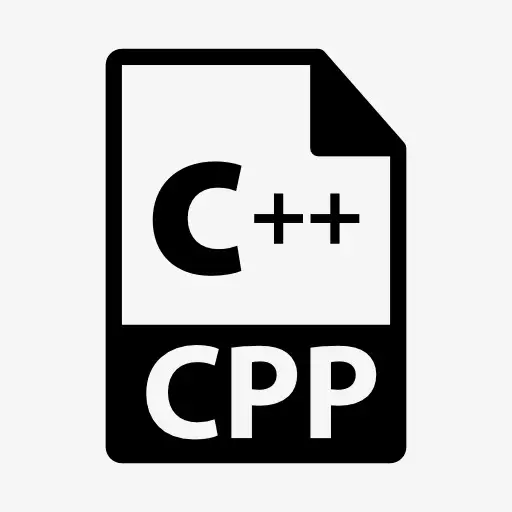
Exercises
Exercise 1-1
Are the following definitions valid? Why or why not?
const std::string hello = "Hello";
const std::string message = hello + ", world" + "!";
编译正确。std::string 重写了 + 操作符
Exercise 1-2
Are the following definitions valid? Why or why not?
const std::string exclam = "!";
const std::string message = "Hello" + ", world" + exclam;
编译报错。因为 “Hello” 为 const char *,并没有重载操作符,所以编译报错。
Exercise 1-3.
Is the following program valid? If so, what does it do? If not, why not?
#include <iostream>
#include <string>
int main() {
{
const std::string s = "a string";
std::cout << s << std::endl;
}
{
const std::string s = "another string";
std::cout << s << std::endl;
}
return 0;
}
编译正确。作用域没问题
Exercise 1-4
What about this one? What if we change }} to };} in the third line from the end?
#include <iostream>
#include <string>
int main()
{
{
const std::string s = "a string";
std::cout << s << std::endl;
{
const std::string s = "another string";
std::cout << s << std::endl;
}
}
return 0;
}
编译正确。作用域没问题
Exercise 1-5
Is this program valid? If so, what does it do? If not, say why not, and rewrite it to be valid.
#include <iostream>
#include <string>
int main()
{
{
std::string s = "a string";
{
std::string x = s + ", really";
std::cout << s << std::endl;
}
std::cout << x << std::endl;
}
return 0;
}
编译报错。x 超出作用域范围
Exercise 1-6
What does the following program do if, when it asks you for input, you type two names (for example, Samuel Beckett)? Predict the behavior before running the program, then try it.
#include <iostream>
#include <string>
int main()
{
std::cout << "What is your name? ";
std::string name;
std::cin >> name;
std::cout << "Hello, " << name
<< std::endl
<< "And what is yours? ";
std::cin >> name;
std::cout << "Hello, " << name
<< "; nice to meet you too!" << std::endl;
return 0;
}
如果连续输入两个值,整个程序直接结束
因为 cin 碰到空格或者换行符都会终止。
传入两个值,刚好两次 cin 读取,程序结束。
额外补充
感觉 chapter01 也忒简单了···
学习一下 const 关键字吧
源自 CPlusPlusThings https://github.com/Light-City/CPlusPlusThings
总结一下:
-
const 用于定义常量,不可修改
-
const 和 指针
const char* a = “test”;
char* const a = “test”;如果 const 位于
*
的左侧,则 const 就是用来修饰指针所指向的变量,即指针指向为常量;
如果 const 位于*
的右侧,const 就是修饰指针本身,即指针本身是常量。
const 优先作用于左边,左边没有信息,才会作用于右边
int a = 1;
int b = 2;
// 指针 p1 本身是常量指针,指针本身的值不可以修改,但是指针指向的内存区域的值随意修改
int *const p1 = &a;
// p1 = &b; // error
*p1 = 3; // ok
// 指针 p2 指向的内容为常量,指针本身的值可以修改,指针指向的内容区域的值不可修改
const int *p2 = &a;
p2 = &b; // ok
// *p2 = 3; // error
- 函数中的 const
一般作用于参数限制。如传参数递引用时,害怕被修改,通过增加 const 保证不被修改